Question 1: What is JavaScript, and How Does it Differ from Java?
JavaScript, commonly abbreviated as JS, is a versatile, high-level, dynamically-typed, and interpreted scripting language used primarily for web development. It differs significantly from Java, despite the similar name. Java is a statically-typed, compiled language used for a wide range of applications, including server-side programming, mobile app development, and more. In contrast, JavaScript is primarily used for client-side scripting within web browsers to create dynamic and interactive web pages.
Question 2: What are Closures in JavaScript, and Why Are They Useful?
Closures in JavaScript are functions that "remember" their lexical scope, even when they are executed outside that scope. They are useful for data encapsulation, maintaining state, and creating private variables. Here's a more in-depth answer with an example:
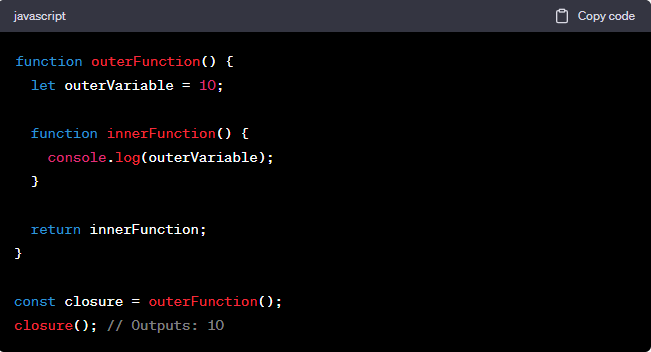
Question 3: What is the Event Loop in JavaScript, and How Does it Work?
The event loop is a critical concept for understanding JavaScript's asynchronous nature. It manages the execution of code, ensuring that non-blocking tasks are handled efficiently. The event loop coordinates the call stack, callback queue, and message queue. It's particularly vital for handling asynchronous operations like fetching data from APIs or handling user interactions.
Question 4: Explain the Difference Between null and undefined in JavaScript.
Understanding the distinctions between null and undefined is essential in JavaScript.
- null represents the intentional absence of any object value. It's often assigned by developers.
- undefined is the default value of a variable that has been declared but not yet assigned a value.
Here's a code example:
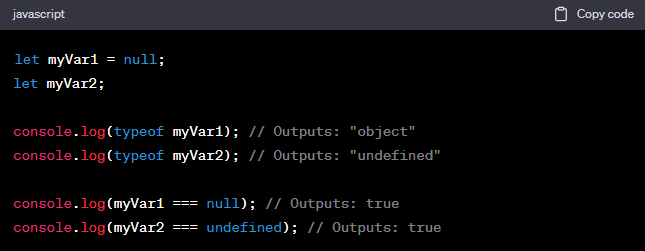
Question 5: What is the Role of Promises in JavaScript, and How Do They Work?
Promises simplify asynchronous programming in JavaScript. They represent a value that may not be available yet. Promises have three states: pending, resolved, and rejected. You can create, chain, and handle Promises using methods like .then() and .catch(). Here's a basic example:
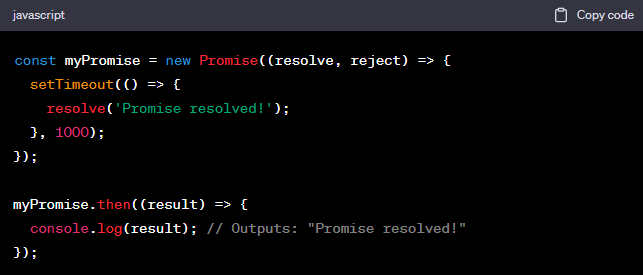
Question 6: Explain the Concept of Hoisting in JavaScript.
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during compilation. However, only the declarations are hoisted, not the initializations. Here's an example:

Question 7: What are Arrow Functions, and How Do They Differ from Regular Functions?
Arrow functions are a concise way to write functions in JavaScript. They have a shorter syntax compared to regular functions and do not have their own this value. Arrow functions are often used for simpler, one-liner functions. Here's a comparison:
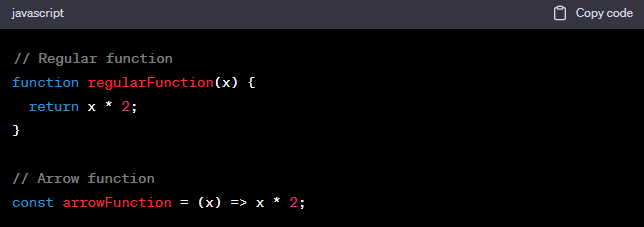
Question 8: Explain the Prototype Chain in JavaScript and How Prototypal Inheritance Works.
JavaScript uses prototypal inheritance, where objects inherit properties and methods from their prototypes. Objects are linked together through their prototypes, forming a prototype chain. Understanding this concept is crucial for working with JavaScript's object-oriented features.
Question 9: What is the Difference Between '==' and '===' Operators in JavaScript?
In JavaScript, '==' is the loose equality operator, while '===' is the strict equality operator. '==' performs type coercion, meaning it converts operands to the same type before comparing. '===' compares both value and type without coercion. Here's an example:

Question 10: How Can You Avoid Callback Hell (Pyramid of Doom) in JavaScript?
Callback hell occurs when you have multiple nested callbacks, making the code difficult to read and maintain. You can mitigate this by using techniques like Promises, async/await, or modularizing your code into smaller, reusable functions.
This article has explored ten crucial JavaScript interview questions, ranging from fundamental concepts to more advanced topics. Mastery of these concepts will enhance your confidence in JavaScript interviews. Remember to practice, and consult additional resources for comprehensive interview preparation.
Conclusion
In conclusion, mastering the fundamental concepts and advanced topics of JavaScript is essential for success in interviews and web development. By delving into closures, event loops, promises, and more, you can enhance your skills and confidence in using this versatile scripting language to create dynamic and interactive web experiences.